Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 면접을 위한 CS 전공 지식 노트 Tree
- 양궁대회
- 롤케이크 자르기
- retain cycle
- 면접을 위한 CS전공 지식 노트
- @escaping
- 테이블뷰 나누기
- UIKit
- CoreData
- CarouselCollectionview
- Reference Cycle
- TableView Section
- UserDefaults
- 자료구조
- Array vs Linked List
- firebase
- TableView
- ReferceCycle
- SWIFT
- class struct
- Input Output
- NavigationSearchBar
- 강한 참조 순환
- 프로그래머스
- Value Type Reference Type
- tableview section별 다른 cell적용
- coremotion
- Carousel CollectionView
- wil
- til
Archives
- Today
- Total
개발하는 동글 :]
[TIL],[UIKit],[FireBase 사용해보기!] 본문
1. FireBase 연결하기
1.1 Firebase 프로젝트 생성하기
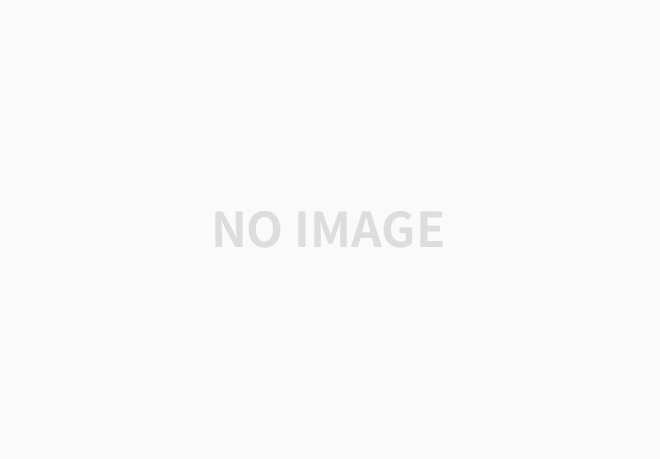
1.2 iOS 버튼을 눌러서 App 등록하기
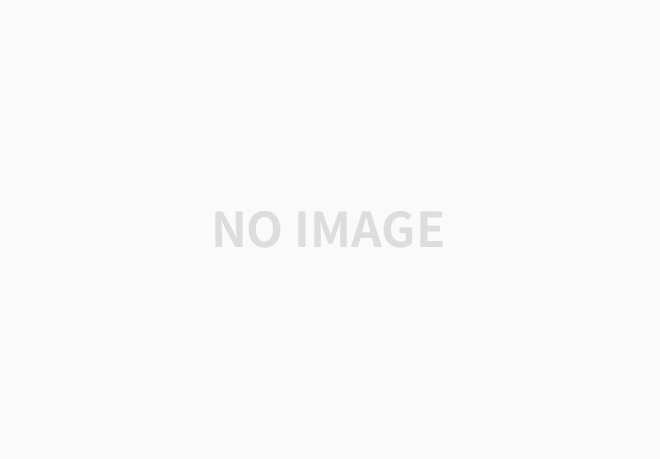
1.3 app등록하기
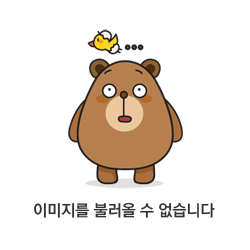
1.4 SPM에서 FireBase 패키지 추가
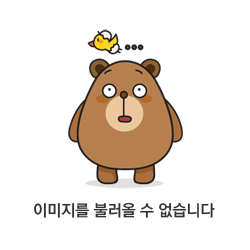
1.5 GoogleService - Info 파일 추가
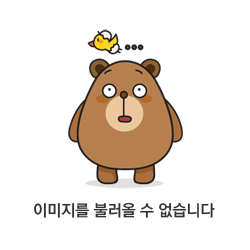
1.6 초기화 코드 추가
import UIKit
import FirebaseCore
@main
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
FirebaseApp.configure()
return true
}
// MARK: UISceneSession Lifecycle
func application(_ application: UIApplication, configurationForConnecting connectingSceneSession: UISceneSession, options: UIScene.ConnectionOptions) -> UISceneConfiguration {
return UISceneConfiguration(name: "Default Configuration", sessionRole: connectingSceneSession.role)
}
func application(_ application: UIApplication, didDiscardSceneSessions sceneSessions: Set<UISceneSession>) {
}
}
2. Firebase Data Manager로 CRUD 구현해 보기!
//
// FireabaseDataManager.swift
// testProject
//
// Created by SeoJunYoung on 10/16/23.
//
import Foundation
import FirebaseCore
import FirebaseFirestore
import FirebaseFirestoreSwift
struct TestData: Codable {
var first: String
var second: String
var three: String
}
struct FBDataManager {
let db = Firestore.firestore()
func create(completion: @escaping () -> Void) {
db.collection("users").addDocument(
data:[
"first" : "1",
"second" : "2",
"three" : "3"
]) { error in
completion()
}
}
func read(completion: @escaping ([TestData]) -> Void) {
db.collection("users").getDocuments {data, error in
guard let datas = data?.documents else { return }
var temp:[TestData] = []
for data in datas {
do {
let res = try Firestore.Decoder().decode(TestData.self, from: data.data())
temp.append(res)
} catch {
print(error)
}
}
completion(temp)
}
}
}